Trigger functions automatically with Stedi Core events and custom schedules
Jul 12, 2023
Products
We recently launched Stedi Core, an event-driven EDI system that does most of the heavy lifting for EDI integrations. Core can validate, parse, and generate EDI for any trading partner and provides complete visibility into your real-time transaction data.
After configuring Core, you can use Stedi Functions to create an end-to-end flow between Core and your internal systems and business applications. Functions can react to Core events to run custom code. You can transform the data shape, call out to external applications and APIs, or extend Stedi to fulfill any requirement.
We want to showcase two features you can use to automatically invoke functions in your EDI integration: event bindings and function schedules.
Event bindings
Core emits events for every file, functional group, and transaction set it processes successfully. Core also emits events for processing failures. Visit the Core events documentation for details.
You can configure event bindings on a function so the function is automatically invoked in response to specific Core events.
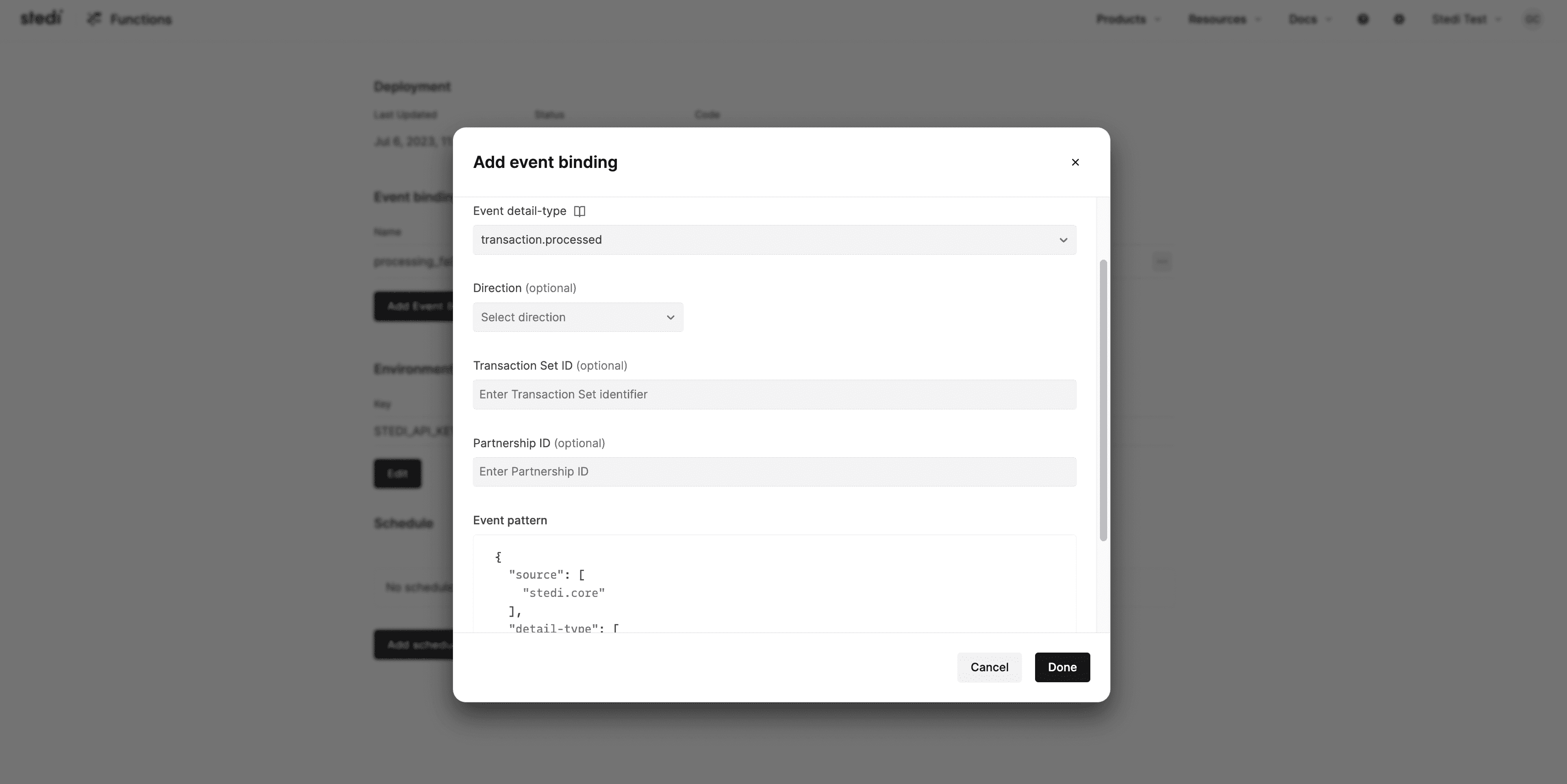
Example: Send inbound purchase orders to an ERP system
The following function has an event binding that listens to transaction.processed
events for inbound 850 Purchase Order transactions from a specific trading partner. When Core successfully processes an 850 transaction, it fires an event. The event binding invokes this function when the filter criteria are met.
The function uses a Stedi mapping to transform the translated JSON output from Core to the JSON shape required by the ERP system. It then calls the ERP system’s API and sends the transformed payload to create the purchase order within the system.
const buckets = bucketsClient();
const mappings = mappingsClient();
export const handler = async (event) => {
const transactionObject = await buckets.getObject({
bucketName: event.detail.output.bucketName,
key: event.detail.output.key,
});
const content = JSON.parse(await transactionObject.body?.transformToString());
const erpJson = await mappings.mapDocument({
id: "<your_mapping_id>",
content,
validationMode: "strict",
});
await fetch(process.env.ERP_CREATE_PURCHASE_ORDER, {
method: "POST",
body: JSON.stringify(erpJson),
headers: {
"Authorization": `Key ${process.env.ERP_API_KEY}`,
"Content-Type": "application/json",
},
});
};
You can find additional example code on GitHub.
Example: Publish processing failures to Slack
Stedi Core emits file.failed
events when it cannot process an inbound file or when it cannot deliver a generated outbound file to a partner. For example, Core emits a file.failed
event when your partner sends an invalid EDI file.
The following function has an event binding that listens to file.failed
events and posts a Slack webhook to notify a support team to take action. You can use this approach to integrate with your own alerting system.
export const handler = async (event) => {
const lines = [
`ISA IDs: receiver: ${event.interchange.receiverId},
sender: ${event.interchange.senderId}`,
`File ID: ${event.fileId}`,
`Direction: ${event.direction}`,
...event.errors.map((err) => `Error: ${err}`),
];
await fetch(process.env["SLACK_URL"], {
method: "POST",
body: JSON.stringify({
blocks: [
{
type: "header",
text: {
type: "plain_text",
text: "⚠️ Stedi Core File Processing Failed",
emoji: true,
},
},
...lines.map((line) => ({
type: "section",
text: {
type: "mrkdwn",
text: line,
},
})),
],
}),
});
};
You can find full template code on GitHub.
Custom schedules
You can quickly set up a basic schedule in the Functions UI to run your function periodically at a set frequency (every X minutes, hours, or days). You can also define advanced schedules with custom expressions to set the frequency of execution, such as specific dates and times in a week.

Example: Poll for inventory data
The following function polls to check inventory levels for product SKUs. When products run low on inventory, the function automatically generates an 850 purchase order based on current stock and preferred vendor information.
Stedi Core uses Stedi guides to generate EDI according to partner-specific requirements. Each guide’s JSON schema represents the shape of the EDI transaction set that the guide defines. This example uses a Stedi mapping to transform the JSON inventory payload into the required schema for 850 purchase orders.
const mappings = mappingsClient();
const core = coreClient();
export const handler = async () => {
const lowInventoryItems = await fetch(process.env.ERP_LOW_INVENTORY_URL, {
method: "GET",
headers: {
Authorization: `Key ${process.env.ERP_API_KEY}`,
},
});
// Use Stedi mapping to transform JSON schema
const lowInventoryOrdersPromises = (await lowInventoryItems.json()).map(async (item) => {
const orderJson = await mappings.mapDocument({
id: "<your_mapping_id>",
content: item,
validationMode: "strict",
});
return core.generateEdi({
partnershipId: orderJson.partnershipId,
transactionGroups: [
{
transactionSettingsId: "004010-850",
transactions: orderJson.transactions,
},
],
});
});
await Promise.all(lowInventoryOrdersPromises);
};
Complete your integration with Stedi Functions
Functions allow you to extend Stedi’s event-driven architecture to fulfill any requirement and build end-to-end EDI integrations tailored to your business.
Book a demo with our onboarding team, and we'll help you set up Stedi Core for your first trading partner and create the functions you need to complete your integration.
We recently launched Stedi Core, an event-driven EDI system that does most of the heavy lifting for EDI integrations. Core can validate, parse, and generate EDI for any trading partner and provides complete visibility into your real-time transaction data.
After configuring Core, you can use Stedi Functions to create an end-to-end flow between Core and your internal systems and business applications. Functions can react to Core events to run custom code. You can transform the data shape, call out to external applications and APIs, or extend Stedi to fulfill any requirement.
We want to showcase two features you can use to automatically invoke functions in your EDI integration: event bindings and function schedules.
Event bindings
Core emits events for every file, functional group, and transaction set it processes successfully. Core also emits events for processing failures. Visit the Core events documentation for details.
You can configure event bindings on a function so the function is automatically invoked in response to specific Core events.
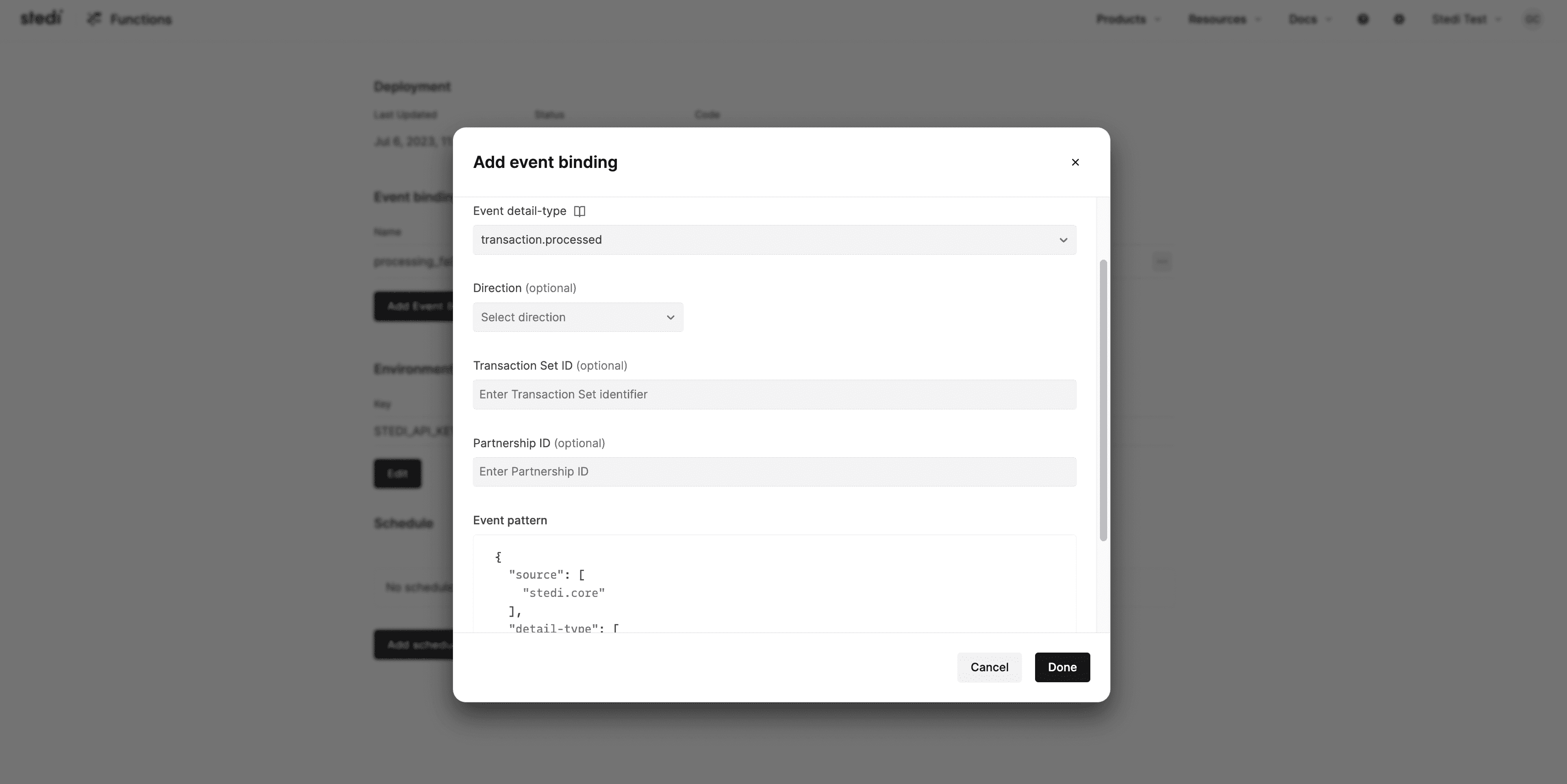
Example: Send inbound purchase orders to an ERP system
The following function has an event binding that listens to transaction.processed
events for inbound 850 Purchase Order transactions from a specific trading partner. When Core successfully processes an 850 transaction, it fires an event. The event binding invokes this function when the filter criteria are met.
The function uses a Stedi mapping to transform the translated JSON output from Core to the JSON shape required by the ERP system. It then calls the ERP system’s API and sends the transformed payload to create the purchase order within the system.
const buckets = bucketsClient();
const mappings = mappingsClient();
export const handler = async (event) => {
const transactionObject = await buckets.getObject({
bucketName: event.detail.output.bucketName,
key: event.detail.output.key,
});
const content = JSON.parse(await transactionObject.body?.transformToString());
const erpJson = await mappings.mapDocument({
id: "<your_mapping_id>",
content,
validationMode: "strict",
});
await fetch(process.env.ERP_CREATE_PURCHASE_ORDER, {
method: "POST",
body: JSON.stringify(erpJson),
headers: {
"Authorization": `Key ${process.env.ERP_API_KEY}`,
"Content-Type": "application/json",
},
});
};
You can find additional example code on GitHub.
Example: Publish processing failures to Slack
Stedi Core emits file.failed
events when it cannot process an inbound file or when it cannot deliver a generated outbound file to a partner. For example, Core emits a file.failed
event when your partner sends an invalid EDI file.
The following function has an event binding that listens to file.failed
events and posts a Slack webhook to notify a support team to take action. You can use this approach to integrate with your own alerting system.
export const handler = async (event) => {
const lines = [
`ISA IDs: receiver: ${event.interchange.receiverId},
sender: ${event.interchange.senderId}`,
`File ID: ${event.fileId}`,
`Direction: ${event.direction}`,
...event.errors.map((err) => `Error: ${err}`),
];
await fetch(process.env["SLACK_URL"], {
method: "POST",
body: JSON.stringify({
blocks: [
{
type: "header",
text: {
type: "plain_text",
text: "⚠️ Stedi Core File Processing Failed",
emoji: true,
},
},
...lines.map((line) => ({
type: "section",
text: {
type: "mrkdwn",
text: line,
},
})),
],
}),
});
};
You can find full template code on GitHub.
Custom schedules
You can quickly set up a basic schedule in the Functions UI to run your function periodically at a set frequency (every X minutes, hours, or days). You can also define advanced schedules with custom expressions to set the frequency of execution, such as specific dates and times in a week.

Example: Poll for inventory data
The following function polls to check inventory levels for product SKUs. When products run low on inventory, the function automatically generates an 850 purchase order based on current stock and preferred vendor information.
Stedi Core uses Stedi guides to generate EDI according to partner-specific requirements. Each guide’s JSON schema represents the shape of the EDI transaction set that the guide defines. This example uses a Stedi mapping to transform the JSON inventory payload into the required schema for 850 purchase orders.
const mappings = mappingsClient();
const core = coreClient();
export const handler = async () => {
const lowInventoryItems = await fetch(process.env.ERP_LOW_INVENTORY_URL, {
method: "GET",
headers: {
Authorization: `Key ${process.env.ERP_API_KEY}`,
},
});
// Use Stedi mapping to transform JSON schema
const lowInventoryOrdersPromises = (await lowInventoryItems.json()).map(async (item) => {
const orderJson = await mappings.mapDocument({
id: "<your_mapping_id>",
content: item,
validationMode: "strict",
});
return core.generateEdi({
partnershipId: orderJson.partnershipId,
transactionGroups: [
{
transactionSettingsId: "004010-850",
transactions: orderJson.transactions,
},
],
});
});
await Promise.all(lowInventoryOrdersPromises);
};
Complete your integration with Stedi Functions
Functions allow you to extend Stedi’s event-driven architecture to fulfill any requirement and build end-to-end EDI integrations tailored to your business.
Book a demo with our onboarding team, and we'll help you set up Stedi Core for your first trading partner and create the functions you need to complete your integration.
We recently launched Stedi Core, an event-driven EDI system that does most of the heavy lifting for EDI integrations. Core can validate, parse, and generate EDI for any trading partner and provides complete visibility into your real-time transaction data.
After configuring Core, you can use Stedi Functions to create an end-to-end flow between Core and your internal systems and business applications. Functions can react to Core events to run custom code. You can transform the data shape, call out to external applications and APIs, or extend Stedi to fulfill any requirement.
We want to showcase two features you can use to automatically invoke functions in your EDI integration: event bindings and function schedules.
Event bindings
Core emits events for every file, functional group, and transaction set it processes successfully. Core also emits events for processing failures. Visit the Core events documentation for details.
You can configure event bindings on a function so the function is automatically invoked in response to specific Core events.
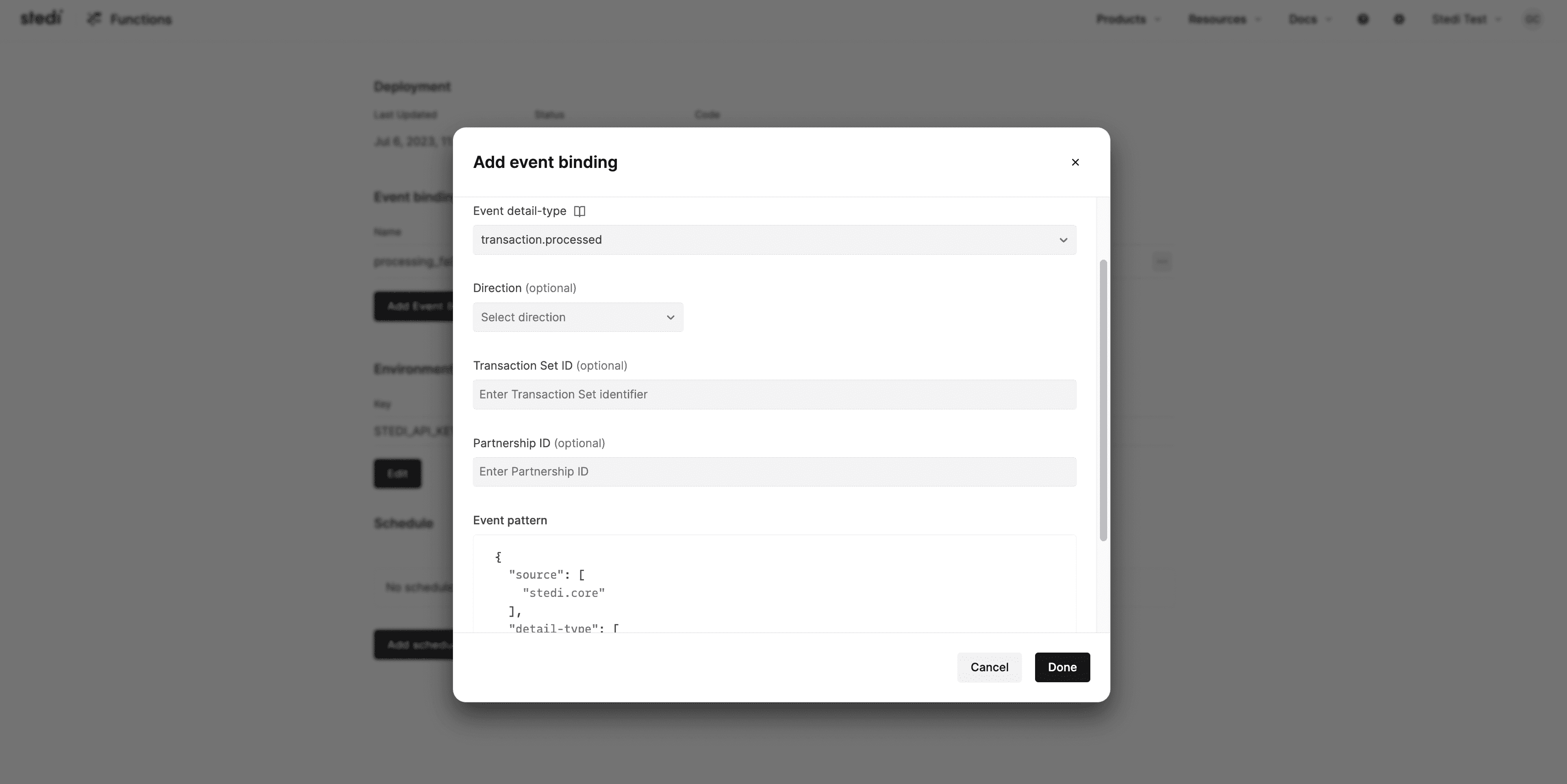
Example: Send inbound purchase orders to an ERP system
The following function has an event binding that listens to transaction.processed
events for inbound 850 Purchase Order transactions from a specific trading partner. When Core successfully processes an 850 transaction, it fires an event. The event binding invokes this function when the filter criteria are met.
The function uses a Stedi mapping to transform the translated JSON output from Core to the JSON shape required by the ERP system. It then calls the ERP system’s API and sends the transformed payload to create the purchase order within the system.
const buckets = bucketsClient();
const mappings = mappingsClient();
export const handler = async (event) => {
const transactionObject = await buckets.getObject({
bucketName: event.detail.output.bucketName,
key: event.detail.output.key,
});
const content = JSON.parse(await transactionObject.body?.transformToString());
const erpJson = await mappings.mapDocument({
id: "<your_mapping_id>",
content,
validationMode: "strict",
});
await fetch(process.env.ERP_CREATE_PURCHASE_ORDER, {
method: "POST",
body: JSON.stringify(erpJson),
headers: {
"Authorization": `Key ${process.env.ERP_API_KEY}`,
"Content-Type": "application/json",
},
});
};
You can find additional example code on GitHub.
Example: Publish processing failures to Slack
Stedi Core emits file.failed
events when it cannot process an inbound file or when it cannot deliver a generated outbound file to a partner. For example, Core emits a file.failed
event when your partner sends an invalid EDI file.
The following function has an event binding that listens to file.failed
events and posts a Slack webhook to notify a support team to take action. You can use this approach to integrate with your own alerting system.
export const handler = async (event) => {
const lines = [
`ISA IDs: receiver: ${event.interchange.receiverId},
sender: ${event.interchange.senderId}`,
`File ID: ${event.fileId}`,
`Direction: ${event.direction}`,
...event.errors.map((err) => `Error: ${err}`),
];
await fetch(process.env["SLACK_URL"], {
method: "POST",
body: JSON.stringify({
blocks: [
{
type: "header",
text: {
type: "plain_text",
text: "⚠️ Stedi Core File Processing Failed",
emoji: true,
},
},
...lines.map((line) => ({
type: "section",
text: {
type: "mrkdwn",
text: line,
},
})),
],
}),
});
};
You can find full template code on GitHub.
Custom schedules
You can quickly set up a basic schedule in the Functions UI to run your function periodically at a set frequency (every X minutes, hours, or days). You can also define advanced schedules with custom expressions to set the frequency of execution, such as specific dates and times in a week.

Example: Poll for inventory data
The following function polls to check inventory levels for product SKUs. When products run low on inventory, the function automatically generates an 850 purchase order based on current stock and preferred vendor information.
Stedi Core uses Stedi guides to generate EDI according to partner-specific requirements. Each guide’s JSON schema represents the shape of the EDI transaction set that the guide defines. This example uses a Stedi mapping to transform the JSON inventory payload into the required schema for 850 purchase orders.
const mappings = mappingsClient();
const core = coreClient();
export const handler = async () => {
const lowInventoryItems = await fetch(process.env.ERP_LOW_INVENTORY_URL, {
method: "GET",
headers: {
Authorization: `Key ${process.env.ERP_API_KEY}`,
},
});
// Use Stedi mapping to transform JSON schema
const lowInventoryOrdersPromises = (await lowInventoryItems.json()).map(async (item) => {
const orderJson = await mappings.mapDocument({
id: "<your_mapping_id>",
content: item,
validationMode: "strict",
});
return core.generateEdi({
partnershipId: orderJson.partnershipId,
transactionGroups: [
{
transactionSettingsId: "004010-850",
transactions: orderJson.transactions,
},
],
});
});
await Promise.all(lowInventoryOrdersPromises);
};
Complete your integration with Stedi Functions
Functions allow you to extend Stedi’s event-driven architecture to fulfill any requirement and build end-to-end EDI integrations tailored to your business.
Book a demo with our onboarding team, and we'll help you set up Stedi Core for your first trading partner and create the functions you need to complete your integration.
Share
Get started with Stedi
Get started with Stedi
Automate healthcare transactions with developer-friendly APIs that support thousands of payers. Contact us to learn more and speak to the team.
Get updates on what’s new at Stedi
Get updates on what’s new at Stedi
Get updates on what’s new at Stedi
Get updates on what’s new at Stedi
Backed by
Stedi is a registered trademark of Stedi, Inc. All names, logos, and brands of third parties listed on our site are trademarks of their respective owners (including “X12”, which is a trademark of X12 Incorporated). Stedi, Inc. and its products and services are not endorsed by, sponsored by, or affiliated with these third parties. Our use of these names, logos, and brands is for identification purposes only, and does not imply any such endorsement, sponsorship, or affiliation.
Get updates on what’s new at Stedi
Backed by
Stedi is a registered trademark of Stedi, Inc. All names, logos, and brands of third parties listed on our site are trademarks of their respective owners (including “X12”, which is a trademark of X12 Incorporated). Stedi, Inc. and its products and services are not endorsed by, sponsored by, or affiliated with these third parties. Our use of these names, logos, and brands is for identification purposes only, and does not imply any such endorsement, sponsorship, or affiliation.
Get updates on what’s new at Stedi
Backed by
Stedi is a registered trademark of Stedi, Inc. All names, logos, and brands of third parties listed on our site are trademarks of their respective owners (including “X12”, which is a trademark of X12 Incorporated). Stedi, Inc. and its products and services are not endorsed by, sponsored by, or affiliated with these third parties. Our use of these names, logos, and brands is for identification purposes only, and does not imply any such endorsement, sponsorship, or affiliation.